Common Intermediate Language (CIL)
Example "untyped instructions"
While Java Bytecode instructions always specify the type of their arguments,
CIL instructions often omit this.
In the example we compare the instructions for loading, adding and storing of
integer and floating-point numbers in CIL and Java Bytecode.
Therefore we use a class Addition with two static methods that
load the values of two local integer or floating-point variables, respectively,
add them and then store the result in a third local variable.
Addition.cs
public class Addition {
public static void AddInt () {
int i1 = 6;
int i2 = 17;
int res = i1 + i2;
}
public static void AddFloat () {
float f1 = 34.4f;
float f2 = 43.1f;
float res = f1 + f2;
}
public static void Main () {
AddInt();
AddFloat();
}
}
|
With the IL disassembler (ildasm.exe ) we can inspect the CIL code of these two methods.
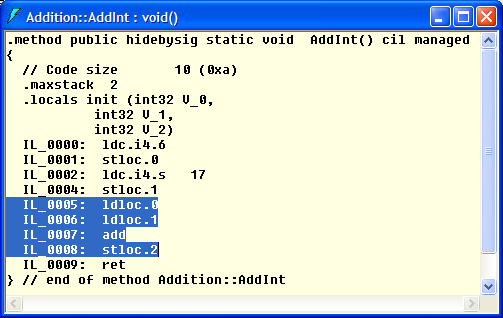
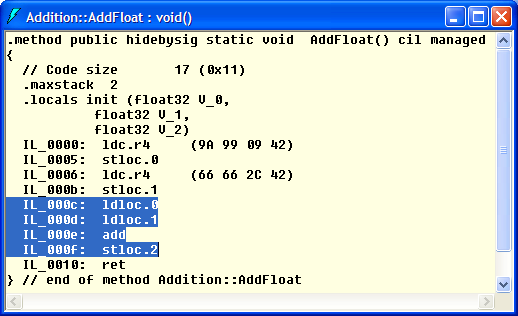
The Java version of class Addition is almost identical to the C# version.
Addition.java
public class Addition {
public static void addInt () {
int i1 = 6;
int i2 = 17;
int res = i1 + i2;
}
public static void addFloat () {
float f1 = 34.4f;
float f2 = 43.1f;
float res = f1 + f2;
}
public static void main (String[] args) {
addInt();
addFloat();
}
}
|
The Java class file disassembler (javap.exe ) shows the Java Bytecode instructions.
>javap Addition -c
Compiled from "Addition.java"
public class Addition extends java.lang.Object{
...
public static void addInt();
Code:
0: bipush 6
2: istore_0
3: bipush 17
5: istore_1
6: iload_0
7: iload_1
8: iadd
9: istore_2
10: return
public static void addFloat();
Code:
0: ldc #2; //float 34.4f
2: fstore_0
3: ldc #3; //float 43.1f
5: fstore_1
6: fload_0
7: fload_1
8: fadd
9: fstore_2
10: return
...
}
Here we can immediately see the different instructions for loading, adding and storing
of integer (i... ) and floating-point (f... ) values in the Java Bytecode.
|